Mình muốn send request có body như sau
[ { "itemId": "abcde32366", "category": 11111, "attributes": { "name": "Quan ao mua dong", "description": "good product", "brand": "30768" }, "skus": [ { "id": "test", "sku": "", "price": 1100000, "quantity": 10 } ] } ]
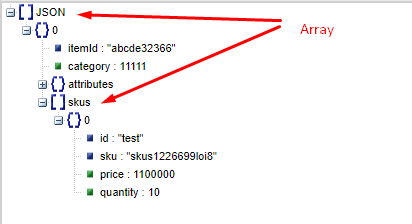
Nội dung bài viết
I. Tạo POJO để mapping

Ở đây, vì lười và vì mình ko muốn tạo nhiều class nên mình sẽ sử dụng nested class như sau:
Lưu ý: ở đây mình tạo ra các constructor có sẵn data để test cho nhanh, trong thực tế các bạn sẽ ko nên hard code như vậy.
import lombok.Data; import java.util.Arrays; import java.util.List; @Data public class Product { private String itemId; private int category; private Attribute attributes; private List<Skus> skus; //Tạo list của Sku public Product() { this.itemId = "abcde32366"; this.category = 11111; this.attributes = new Attribute(); this.skus = Arrays.asList(new Skus()); //Tạo list của Sku } @Data public static class Attribute { private String name; private String description; private String brand; public Attribute() { this.name = "Quan ao mua dong"; this.description = "good product"; this.brand = "30768"; } } @Data public static class Skus { private String id; private String sku; private long price; private int quantity; public Skus() { this.id = "test"; this.sku = ""; this.price = 1100000; this.quantity = 10; } } }
II. Viết Test
public class ProductTest { @Test void name() { Product product = new Product(); given().log().all().contentType(ContentType.JSON) .body(Arrays.asList(product)) (1) .post("https://postman-echo.com/post") .prettyPrint(); } }
(1) Điểm quan trọng là phải tạo ra 1 list product
cho body, mình sẽ làm điều đó thông qua
- Arrays.asList –> phù hợp cho java 8
- List.of –> phù hợp cho java 11
Request log như sau:
Request method: POST Request URI: https://postman-echo.com/post Proxy: <none> Request params: <none> Query params: <none> Form params: <none> Path params: <none> Headers: Accept=*/* Content-Type=application/json Cookies: <none> Multiparts: <none> Body: [ { "itemId": "abcde32366", "category": 11111, "attributes": { "name": "Quan ao mua dong", "description": "good product", "brand": "30768" }, "skus": [ { "id": "test", "sku": "", "price": 1100000, "quantity": 10 } ] } ]